はじめに
https://astaxie.gitbooks.io/build-web-application-with-golang/ja/
を読書してWebアプリケーションを作成する備忘録を記載していきます。
環境
Windows 10 Professional
Visual Studio Code 1.41.1
Go 1.13.5
Goの環境設定
Goのインストール
基本的なセットアップは済ませているので、サラッと読み流します。
※私の場合は chocolately
という Windowsのパッケージマネージャーのソフト(サードパーティ)を使用してインストールしています。choco install golang
とかでいけるはずです。
GOPATHとワーキングディレクトリ
Go Modules
を使用すると $GOPATH
の中で作業しないでよしなにしてくれるらしいので、これを使用します。
- ワーキングディレクトリの作成
D:/workspace_vscode/building-web-application-with-golang
- 初期設定
go mod init k-bushil.com/webapp
Go Modulesの設定をします。go.mod
が作成されます。
sqrt.go
の作成
mkdir mymath
cd $_
touch sqrt.go
sqrt.go
をコピペします。(下記のようになるはずです。)
- コンパイル
mathapp
フォルダを作成して、main.go
を作成し、main.go
をコピペする
package main
import (
"k-bushil.com/webapp/mymath"
"fmt"
)
func main() {
fmt.Printf("Hello, world. Sqrt(2) = %v\n", mymath.Sqrt(2))
}
go mod
で作成しているので、 mymath
=> k-bushi.com/webapp/mymath
になっていることに注意
結果
hiroto@DESKTOP-M2SDB0M MINGW64 /d/workspace_vscode/building-web-application-with-golang/mathapp
$ go build
hiroto@DESKTOP-M2SDB0M MINGW64 /d/workspace_vscode/building-web-application-with-golang/mathapp
$ ./mathapp.exe
Hello, world. Sqrt(2) = 1.414213562373095
- リモートパッケージの取得
cd /d/workspace_vscode/building-web-application-with-golang/
go get github.com/astaxie/beedb
go.mod
が以下のように、 require
の部分が追加されている。
module k-bushil.com/webapp
go 1.13
require github.com/astaxie/beedb v0.0.0-20141221130223-1732292dfde4 // indirect
go.sum
が新しくできてました。
確認してみると、下記のように $GOPATH
配下に mod/github.com...
と今回 go get
したパッケージが入っていました。
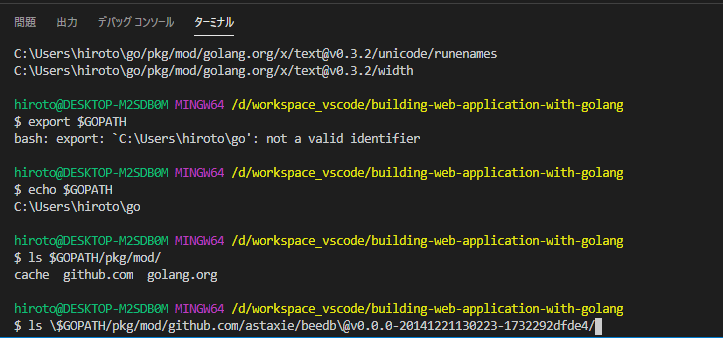
import "github.com/astaxie/beedb"
でインポートできるようです。
Go Modules
を使用しているので、フォルダ構成は src
配下のみと考えるのが良さそうです。
Goのコマンド
ここは解説メインの章っぽいので、 ローカルの go
コマンドを叩いたときの出力を記載しておきます。
$ go
Go is a tool for managing Go source code.
Usage:
go <command> [arguments]
The commands are:
bug start a bug report
build compile packages and dependencies
clean remove object files and cached files
doc show documentation for package or symbol
env print Go environment information
fix update packages to use new APIs
fmt gofmt (reformat) package sources
generate generate Go files by processing source
get add dependencies to current module and install them
install compile and install packages and dependencies
list list packages or modules
mod module maintenance
run compile and run Go program
test test packages
tool run specified go tool
version print Go version
vet report likely mistakes in packages
Use "go help <command>" for more information about a command.
Additional help topics:
buildmode build modes
c calling between Go and C
cache build and test caching
environment environment variables
filetype file types
go.mod the go.mod file
gopath GOPATH environment variable
gopath-get legacy GOPATH go get
goproxy module proxy protocol
importpath import path syntax
modules modules, module versions, and more
module-get module-aware go get
module-auth module authentication using go.sum
module-private module configuration for non-public modules
packages package lists and patterns
testflag testing flags
testfunc testing functions
Use "go help <topic>" for more information about that topic.
gofmt
に関してはメモしておきます。(以下引用となります。)
-l
フォーマットする必要のあるファイルを表示します。-w
修正された内容を標準出力に書き出すのではなく、直接そのままファイルに書き込みます。-r
“a[b:len(a)] -> a[b:]”のような重複したルールを追加します。大量に変換を行う際に便利です。-s
ファイルのソースコードを簡素化します。-d
ファイルに書き込まず、フォーマット前後のdiffを表示します。デフォルトはfalseです。-e
全ての文法エラーを標準出力に書き出します。もしこのラベルを使わなかった場合は異なる10行のエラーまでしか表示しません。-cpuprofile
テストモードをサポートします。対応するするcpufile指定のファイルに書き出します。
go fmt
= gofmt -l -w
とすれば修正すべきファイルに直接修正内容を書き込んでくれるらしい。
go fmt mathapp/main.go
とかやってみると実際に修正されました。
Goの開発ツール
VisualStudioCodeを使ってやっていきます。
次回
2章をやります。