はじめに
Nuxt.js
の環境を作成し、VisualStudioCode
でVue
, Nuxt.js
を使用する環境を設定します。
前回の PHP の記事と同様に、コード補完やコード整形のツールをインストールすることで、開発効率やチーム開発の際に統一されたコードを構築することができます。
環境
1
2
3
4
| Windows 10 Professional
Visual Studio Code 1.52.1
node 15.6.0
npm 7.4.0
|
Nuxt.js のプロジェクトのセットアップ
必要なソフトのセットアップ
node + npm のインストール
前提条件
Chocolately
がインストールされていること
- 管理者権限でコマンドプロンプトを実行します。
- 下記コマンドで
nodist
(node
の様々なバージョンを切り替えられるソフト)を入れます。
Node
のバージョンを切り替えます。
を実行すると、node
のバージョンが一覧として出てきます。
今回は最新の、 v15.6.0
を選びます。
を実行します。
4. 次に、 npm
のバージョンを切り替えます。
を実行します。
Node
のバージョンによって、npm
のバージョンは異なりますので、下記で確認しましょう。
https://nodejs.org/ja/download/releases/
yarn のインストール
- 下記コマンドで、
yarn
を入れておきます。
1
| npm install --global yarn
|
参考: https://classic.yarnpkg.com/en/docs/install/#windows-stable
Nuxt.js のアプリケーションセットアップ
参考: https://ja.nuxtjs.org/docs/2.x/get-started/installation
- 下記でプロジェクトをセットアップします。
1
| npm init nuxt-app webtools
|
今回は、 <project-name>
の部分に、 webtools
と入れて実行します。
※yarn
のコマンドの方だと、失敗したため npm
でやりました。
2. 対話形式でプロジェクトの構成を聞かれるので、下記画像の通りにインストールしました。
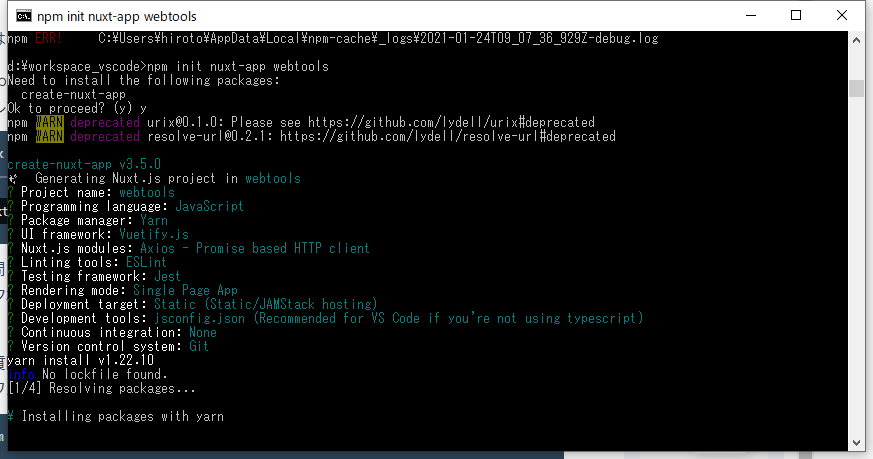
3. 下記コマンドで、インストールできたかを確認します。
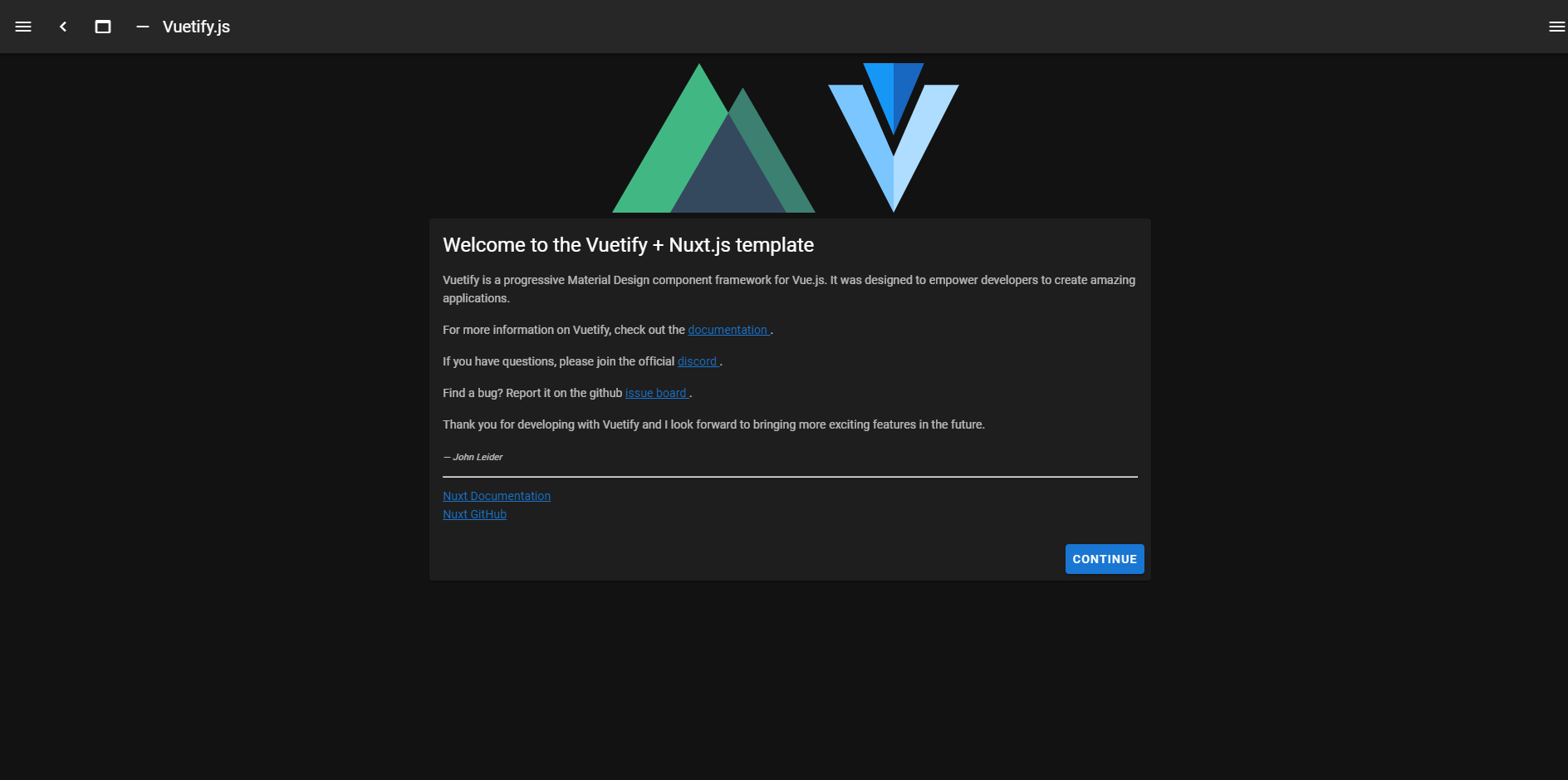
※エラー
yarn dev
を実行後、下記のエラーが出ました。
1
| listen EACCES: permission denied 127.0.0.1:3000
|
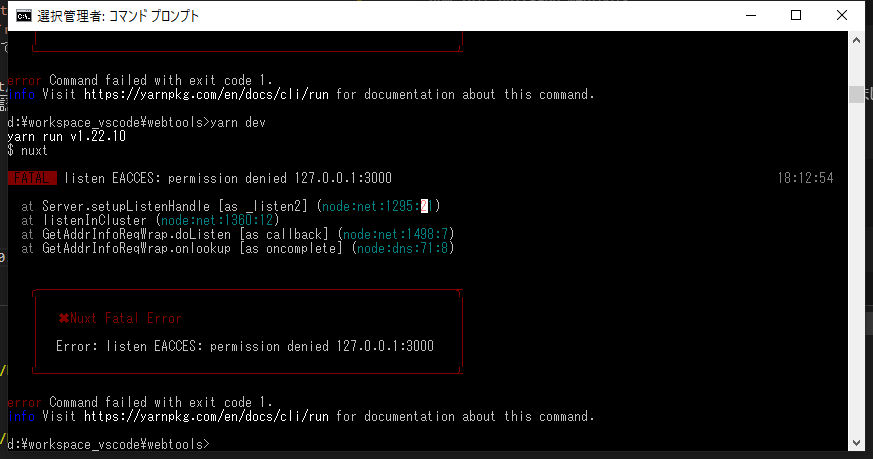
<対処方法>
私の環境ではどうしても原因が見つけられませんでしたので、nuxt.config.js
で開発サーバのポートを変更しました。
下記を追加して、 8001
ポートでサーバを起動するようにしました。
1
2
3
| server: {
port: process.env.NODE_ENV !== 'production' ? 8001 : process.env.PORT
},
|
- 動作確認
Vue.js コード整形+補完ツールのセットアップ
セットアップ
- VisualStudioCode を起動します。
- Ctrl + Shift + P を押してコマンドパレットを開いて、「install extensions」を入力し拡張機能のインストールを選択します。
- 下記をインストールします。
- vetur
*.vue ファイルのシンタックスハイライト - prettier
コード整形 - ESLint
Javascript の静的解析
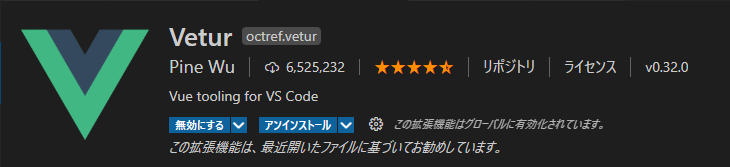
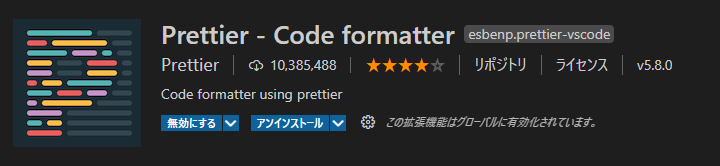
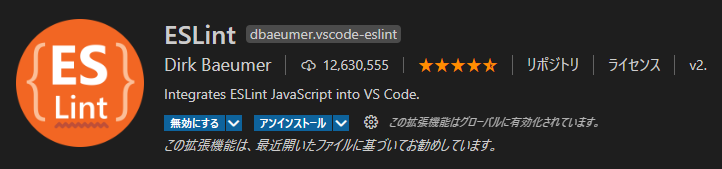
Ctrl + Shift + P を押してコマンドパレットを開いて、「settings」を入力し基本設定(JSON)を開きます。
下記を追記します。
参考にさせていただいたサイト様
https://qiita.com/TigRig/items/36ed8e062d1c32c12b63
https://gurutaka-log.com/nuxt-eslint-prettier#STEP1eslintrcjs
https://wemo.tech/3307
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
| "editor.formatOnSave": false,
// ESLint 自動フォーマット有効化
"eslint.autoFixOnSave": true,
// ESLint の Vue.js 設定
"eslint.validate": [
"vue",
"html",
"javascript",
"typescript",
"javascriptreact",
"typescriptreact"
],
// Prettier の ESLint 連携
"prettier.eslintIntegration": true,
// Vetur のコード整形と Prettier がバッティングしないように無効化
"vetur.format.enable": false
"editor.defaultFormatter": "esbenp.prettier-vscode",
|
- 先ほど作成した、
Nuxt.js
のプロジェクトで下記を入れます。
1
| yarn add --dev prettier eslint-config-prettier eslint-plugin-prettier
|
- 動作確認
Linter
も動いていることが確認できました。
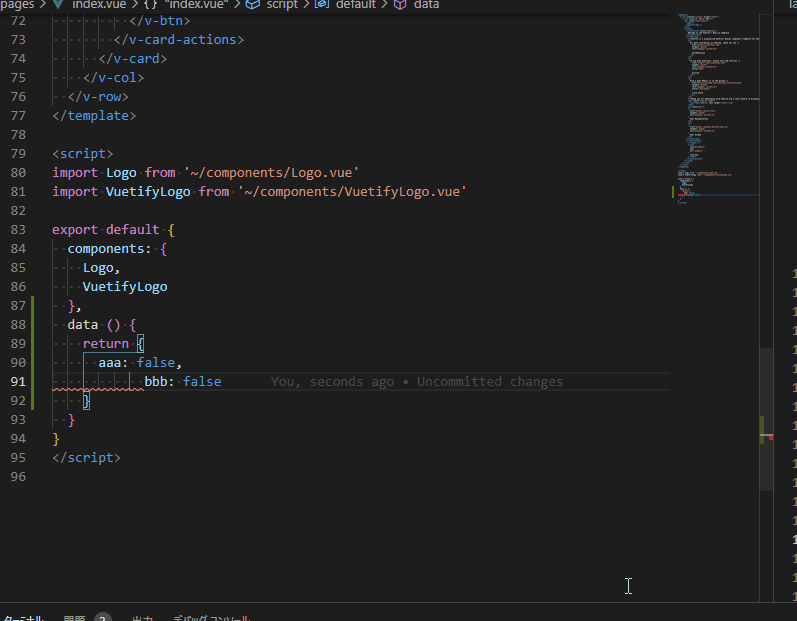
eslintrc.js
, .editorconfig
, jsconfig.json
, .prettierrc
は下記にしています。
.eslintrc.js
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
29
30
| module.exports = {
root: true,
env: {
browser: true,
node: true
},
parserOptions: {
parser: 'babel-eslint'
},
extends: ['@nuxtjs', 'plugin:nuxt/recommended', 'eslint:recommended', 'plugin:prettier/recommended'],
plugins: [],
// add your custom rules here
rules: {
'prettier/prettier': [
'error',
{
htmlWhitespaceSensitivity: 'ignore'
}
],
'vue/html-self-closing': [
'error',
{
html: {
void: 'always'
}
}
],
'vue/singleline-html-element-content-newline': 'off'
}
}
|
.editorconfig
1
2
3
4
5
6
7
8
9
10
11
12
13
14
| ## editorconfig.org
root = true
[*]
indent_style = space
indent_size = 2
end_of_line = lf
charset = utf-8
trim_trailing_whitespace = true
insert_final_newline = true
[*.md]
trim_trailing_whitespace = false
|
jsconfig.json
1
2
3
4
5
6
7
8
9
10
11
12
| {
"compilerOptions": {
"baseUrl": ".",
"paths": {
"~/*": ["./*"],
"@/*": ["./*"],
"~~/*": ["./*"],
"@@/*": ["./*"]
}
},
"exclude": ["node_modules", ".nuxt", "dist"]
}
|
.prettierrc
1
2
3
4
5
6
7
| {
"printWidth": 120,
"tabWidth": 2,
"singleQuote": true,
"trailingComma": "none",
"semi": false
}
|